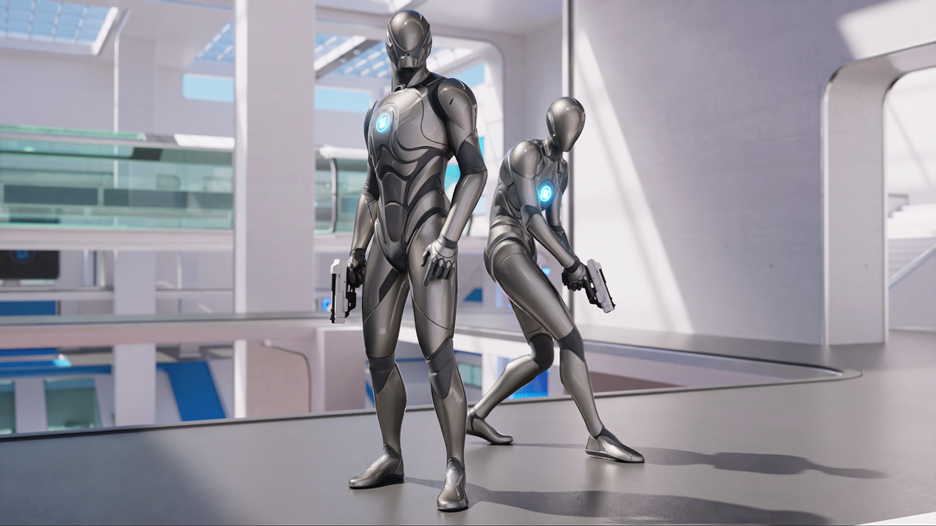
Building dedicated servers is challenging, and developers need the ability to quickly test and iterate on their games. The recently updated Amazon GameLift Server SDK from Amazon Web Services (AWS) provides an Unreal Engine plugin that is compatible with both Unreal Engine 4 and Unreal Engine 5.
The plugin makes it easy for Unreal Engine developers to use an Amazon GameLift Anywhere fleet to test their game server on a local workstation without the need to upload a build to Amazon GameLift. Amazon GameLift Anywhere fleets also integrate with Amazon GameLift FlexMatch and Queues, allowing end-to-end testing.
This post describes how to integrate the Amazon GameLift Server SDK 5.0 plugin into Unreal Engine 5, create an Anywhere fleet, and register your local workstation as a compute resource. You will then create a game session running on your local workstation, saving time and shortening the game development feedback loop.
Solution overview
Unreal Engine 5 offers a free sample game project called Lyra that provides online multiplayer support. This project is a great starting point for building a dedicated server, and in this walkthrough, we integrate the Amazon GameLift plugin into Lyra and describe how to use Amazon GameLift Anywhere to test and iterate on your game server.
Unreal Engine 5 Lyra Starter Game
Walkthrough
This walkthrough takes you through the following steps:
- Build Unreal Engine from source
- Build the Lyra Starter Game
- Set up the Amazon GameLift plugin
- Configure the Lyra Starter Game to use the plugin
- Set up an Amazon GameLift Anywhere fleet
- Create a game session
Prerequisites
For this walkthrough, you should have the following:
- An AWS account
- A GitHub account
- An Epic Games account
- Visual Studio 2022
- OpenSSL and cmake installed
- Prior experience with Unreal Engine and C++
Build Unreal Engine from source
In order to build a dedicated server, you need to compile Unreal Engine from source. This is required so that you can specify a Server Target for the build. Unreal Engine 5.1.0 is used throughout this blog post.
To build your desired version of Unreal Engine
- Follow these steps to link your GitHub account to your Epic Games account.
- Clone your desired version of the Unreal GitHub repo by running:
git clone -b 5.1.0-release --single-branch https://github.com/EpicGames/UnrealEngine.git
- If you plan to cross-compile for Linux, you will need to install the cross-compile toolchain.
- Follow the Unreal Engine documentation to build the engine from source.
Build the Lyra Starter Game
To download Lyra
- Open the Epic Games Launcher
- Click on the Marketplace tab and search for “Lyra”
- Click “Create Project”
- Select your Unreal Engine version and click “Create”
To build Lyra
- Right-click on the
LyraStarterGame
project file and select “Switch Unreal Engine version…”. Select your Unreal source build.
- Right-click on the
LyraStarterGame
project file and select “Generate Visual Studio project files”. - Open Lyra project in the Unreal Editor.
- Follow the dedicated server tutorial in the Unreal documentation to configure Lyra and build, package, and test both a server and a client.
Set up the Amazon GameLift plugin
The Amazon GameLift plugin supports building both Windows and Linux servers. In both cases, the Managed Servers C++ SDK must be compiled, and the library files copied into the plugin folder.
Download the Amazon Gamelift plugin
- Download the latest version of the Amazon GameLift Managed Servers C++ SDK.
- Unzip the archive.
To prepare the plugin for Windows servers
- Open the Visual Studio 2022 Developer Command Prompt.
- Change directory to the C++ SDK folder.
- Build the Windows library by running:
- This creates 2 library files in the outgamelift-server-sdkRelease folder:
aws-cpp-sdk-gamelift-server.lib
aws-cpp-sdk-gamelift-server.dll
- Copy these files into the Amazon GameLift Unreal plugin package in
ThirdPartyGameLiftServerSDKWin64
Prepare the plugin for Linux servers
Optionally, you can build and integrate the Linux version of the library, so that the plugin also supports Linux servers.
- Change directory to the C++ SDK folder.
- Use a machine or a docker container running Amazon Linux 2 to build the Linux version of the library by running:
- This creates a library file
libaws-cpp-sdk-gamelift-server.so in the out/gamelift-server-sdk
folder - Copy this file into the Amazon GameLift Unreal plugin package in:
ThirdPartyGameLiftServerSDKLinuxx86_64-unknown-linux-gnu
Configure the Lyra Starter Game to use the plugin
With the plugin ready to use:
- Enable the plugin in the Lyra Starter Game project
- Add code to use the Amazon GameLift SDK
Enabling the plugin
- Copy the top-level
GameLiftServerSDK
folder into theLyraStarterGamePlugins
folder. The folder structure should look like the following:
- Enable the plugin by editing the LyraStarterGame.uproject file and adding the GameLiftServerSDK plugin to the bottom of the list of plugins:
- Edit the Source
LyraGameLyraGame.Build.cs
file and add “GameLiftServerSDK
” to the list of public module dependencies:
- Open the LyraStarterGame project in Unreal Editor and allow it to rebuild the plugin on startup.
Using the Amazon GameLift Server SDK
When you call InitSDK()
to initialize the Amazon GameLift Server SDK, you need to provide a FServerParameters
object, containing values for how the SDK should be initialized.
With managed Amazon EC2 instances, these server parameter values can be left empty.
However, when setting up an Amazon GameLift Anywhere fleet, you need to specify server parameters to send to Amazon GameLift:
- The Host ID/Compute Name of the Amazon GameLift Anywhere instance
- The Anywhere Fleet ID
- The WebSocket URL
- The Process ID of the running process
- The compute authorization token
When you want to test your server as part of an Amazon GameLift Anywhere fleet, you can provide these parameters via the command line. When you deploy to a managed Amazon EC2 fleet, you can simply omit these parameters.
You will add code to:
- Initialize the Amazon GameLift Server SDK via the InitSDK action, using our command line parameters, if provided.
- Inform the Amazon GameLift service that our process is ready to host players via the ProcessReady action, implementing callback functions to handle requests from the Amazon GameLift service to check the process health, activate a new game session, and terminate a running game session.
- Open SourceLyraGameLyraGameMode.h and add a private function definition for InitGameLift(). Add a definition to override the BeginPlay() protected function:
- Open
SourceLyraGameLyraGameMode.cpp
and include the Amazon GameLift Server SDK header file at the top of the file:
#include “GameLiftServerSDK.h”
- Add the code for the
BeginPlay
andInitGameLift
functions at the bottom of the file:
- Build with a
Development Server
Solution Configuration in Visual Studio 2022.
- Package the server in the Unreal Editor.
Set up an Amazon GameLift Anywhere fleet
Now that you have your dedicated server ready, the next step is to create an Amazon GameLift Anywhere fleet that allows you to place game sessions on your local workstation.
Create an Amazon GameLift Anywhere fleet
- Create a custom location and specify a location name. Custom location names must begin with “custom-“ and must be a minimum of 8 characters in length:
aws gamelift create-location --location-name custom-
- Create a fleet using the newly created location, specifying the compute type as ANYWHERE:
aws gamelift create-fleet --name
- You will be returned some attributes of the fleet – you will need the
FleetId
for the next step.
Register your workstation and start the server
- Register your local workstation as a compute resource in the fleet:
aws gamelift register-compute --compute-name
You will be returned a JSON object with the compute attributes. Make a note of the GameLiftServiceSdkEndpoint
– you will need to pass this in to your game server.
- Obtain a compute authorization token for your local workstation:
aws gamelift get-compute-auth-token --fleet-id
You will be returned a JSON object with an AuthToken which is required to authorize the server with Amazon GameLift when you initialize the Amazon GameLift Server SDK.
NOTE – the AuthToken
expires 15 minutes after creation. Once the token expires, you will need to retrieve a new AuthToken
for a different process.
- Start the Lyra game server, passing in the compute name, the
GameLiftServiceSdkEndpoint
, theAuthToken
and theFleetId
:
LyraServer.exe -log -fleetid=
- You should see logs indicating that the call to
InitSDK
has succeeded andProcessReady
has been successfully called:
If the call to InitSDK
fails, check the command line parameters and make sure you have provided the correct details, and the AuthToken
has not expired.
Create a game session
The final step is to create a game session running on your fleet.
In production, it is always recommended to use game session queues and use game session placement events to track the progress of your queue placement.
In development, you can do this via the create-game-session console command:
aws gamelift create-game-session --fleet-id
You will be returned a JSON object with a Status that will be ACTIVATING if everything worked correctly.
You should also see an entry appear in your game server logs showing that the game server is activating:
You can now run the Lyra Starter game client to connect in to your server:
LyraClient.exe -log
Conclusion
You have now learned to integrate the Amazon GameLift Server SDK into Unreal Engine 5 to start game sessions and carry out local testing via Amazon GameLift Anywhere.
To learn more, please visit these additional resources:
AWS Marketplace | Unreal Engine 5
Amazon GameLift adds support for Unreal Engine 5
Introducing Amazon GameLift Anywhere
Amazon GameLift Anywhere Documentation
Deploying an Anywhere Fleet on Amazon GameLift Tutorial Video
Setting Up Dedicated Servers | Unreal Documentation
Unreal Engine 5 Lyra Starter Game | Unreal Documentation
Multiplayer Programming | Unreal Documentation
https://aws.amazon.com/blogs/gametech/unreal-engine-5-dedicated-server-development-with-amazon-gamelift-anywhere/